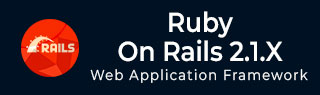
- 学习 Ruby on Rails
- Rails 2.1 主页
- Rails 2.1 简介
- 导轨 2.1 安装
- Rails 2.1 框架
- Rails 2.1 目录结构
- Rails 2.1 示例
- Rails 2.1 数据库设置
- Rails 2.1 活动记录
- Rails 2.1 迁移
- Rails 2.1 控制器
- Rails 2.1 视图
- Rails 2.1 布局
- Rails 2.1 脚手架
- Rails 2.1 和 AJAX
- Rails 2.1 上传文件
- Rails 2.1 发送电子邮件
- 高级 Ruby on Rails 2.1
- Rails 2.1 RMagick 指南
- Rails 2.1 基本 HTTP 身份验证
- Rails 2.1 错误处理
- Rails 2.1 路线系统
- Rails 2.1 单元测试
- 高级 Ruby on Rails 2.1
- Rails 2.1 提示与技巧
- 快速参考指南
- 快速参考指南
- Ruby on Rails 2.1 有用资源
- Ruby on Rails 2.1 - 资源
- Ruby on Rails 2.1 - 讨论
Ruby on Rails 2.1 - 发送电子邮件
ActionMailer是 Rails 组件,使应用程序能够发送和接收电子邮件。在本章中,我们将了解如何使用 Rails 发送电子邮件。
让我们开始使用以下命令创建电子邮件项目。
C:\ruby> rails -d mysql emails
这里我们使用-d mysql选项来指定我们使用 MySQL 数据库的兴趣。我们可以使用-d选项指定任何其他数据库名称,例如oracle或postgress。默认情况下,Rails 使用SQLite数据库。
设置数据库
尽管我们在应用程序中没有使用数据库,但 Rails 需要它才能继续。因此,让我们执行这些附加步骤。
下面给出的是创建数据库的方法 -
mysql> create database emails; Query OK, 1 row affected (0.01 sec) mysql> grant all privileges on emails.* to 'root'@'localhost' identified by 'password'; Query OK, 0 rows affected (0.00 sec) mysql> FLUSH PRIVILEGES; Query OK, 0 rows affected (0.00 sec)
要指示 Rails 找到数据库,请编辑配置文件 ~\upload\config\database.yml 并将数据库名称更改为 Cookbook。完成后,它应该如下所示 -
development: adapter: mysql encoding: utf8 database: emails username: root password: password host: localhost test: adapter: mysql encoding: utf8 database: emails username: root password: password host: localhost production: adapter: mysql encoding: utf8 database: emails username: root password: password host: localhost
操作邮件程序 – 配置
以下是在继续实际工作之前完成配置所必须遵循的步骤。-
转到电子邮件项目的 config 文件夹,打开environment.rb文件,然后在该文件的底部添加以下行。
ActionMailer::Base.delivery_method = :smtp
它通知 ActionMailer 您要使用 SMTP 服务器。如果您使用基于 Unix 的操作系统(例如 Mac OS X 或 Linux),您也可以将其设置为 :sendmail。
还在environment.rb 的底部添加以下代码行。
ActionMailer::Base.smtp_settings = { :address => "smtp.tutorialspoint.com", :port => 25, :domain => "tutorialspoint.com", :authentication => :login, :user_name => "username", :password => "password", }
将每个哈希值替换为简单邮件传输协议 (SMTP) 服务器的正确设置。如果您还不知道,可以从您的互联网服务提供商处获取此信息。如果您使用标准 SMTP 服务器,则无需更改端口号 25 和身份验证类型。
您还可以更改默认电子邮件格式。如果您更喜欢以 HTML 而不是纯文本格式发送电子邮件,请将以下行添加到 config/environment.rb 中 -
ActionMailer::Base.default_content_type = "text/html"
ActionMailer::Base.default_content_type 可以设置为“text/plain”、“text/html”和“text/enriched”。默认值为“文本/纯文本”。
下一步是创建邮件程序。
生成邮件程序
使用以下命令生成邮件程序,如下所示 -
C:\ruby\> cd emails C:\ruby\emails> ruby script/generate mailer Emailer
它将在 app/models 目录中创建一个文件 emailer.rb。检查该文件的内容如下 -
class Emailer < ActionMailer::Base end
现在让我们在 ActionMailer::Base 类中创建一个方法,如下所示 -
class Emailer < ActionMailer::Base def contact(recipient, subject, message, sent_at = Time.now) @subject = subject @recipients = recipient @from = 'no-reply@yourdomain.com' @sent_on = sent_at @body["title"] = 'This is title' @body["email"] = 'sender@yourdomain.com' @body["message"] = message @headers = {} end end
contact 方法有四个参数:收件人、主题、消息和sent_at(定义何时发送电子邮件)。该方法还定义了六个标准参数,它们是每个 ActionMailer 方法的一部分 -
@subject 定义电子邮件主题。
@body 是一个 Ruby 哈希,其中包含可用于填充邮件模板的值。您创建了三个键值对:标题、电子邮件和消息
@recipients 是消息发送对象的列表。
@from 定义电子邮件的发件人。
@sent_on 采用sent_at 参数并设置电子邮件的时间戳。
@headers 是另一个允许您修改电子邮件标头的哈希值。例如,如果您想发送纯文本或 HTML 电子邮件,则可以设置电子邮件的 MIME 类型。
创建控制器
现在,我们将为该应用程序创建一个控制器,如下所示 -
C:\ruby\emails> ruby script/generate controller Emailer
让我们在 app/controllers/emailer_controller.rb 中定义一个控制器方法sendmail,它将调用 Model 方法来发送实际的电子邮件,如下所示 -
class EmailerController < ApplicationController def sendmail recipient = params[:email] subject = params[:subject] message = params[:message] Emailer.deliver_contact(recipient, subject, message) return if request.xhr? render :text => 'Message sent successfully' end end
要使用发件人的联系方法发送电子邮件,您必须将Deliver _ 添加到方法名称的开头。添加 return if request.xhr?,以便在浏览器不支持 JavaScript 时转义到 Rails Java Script (RJS),然后指示该方法呈现文本消息。
除了准备一个屏幕之外,您已基本完成,您可以从中获取用户信息以发送电子邮件。让我们在控制器中定义一个屏幕方法索引,然后在下一节中,我们将定义所有必需的视图 -
在 emailer_controller.rb 文件中添加以下代码。
def index render :file => 'app\views\emailer\index.html.erb' end
定义视图
在 app\views\emails\index.html.erb 中定义视图。这将被称为应用程序的默认页面,并允许用户输入消息并发送所需的电子邮件 -
<h1>Send Email</h1> <% form_tag :action => 'sendmail' do %> <p><label for="email_subject">Subject</label>: <%= text_field 'email', 'subject' %></p> <p><label for="email_recipient">Recipient</label>: <%= text_field 'email', 'recipient' %></p> <p><label for="email_message">Message</label><br/> <%= text_area 'email', 'message' %></p> <%= submit_tag "Send" %> <% end %>
除了上面的视图之外,我们还需要一个模板,该模板将由电子邮件发送者在发送消息时的联系方式使用。这只是带有标准 Rails <%= %> 占位符的文本。
只需将以下代码放入app/views/contact.html.erb文件中即可。
Hi! You are having one email message from <%= @email %> with a title <%= @title %> and following is the message: <%= @message %> Thanks
休息测试
在测试之前,请确保您的计算机已连接到互联网,并且您的电子邮件服务器和网络服务器已启动并正在运行。
现在,使用 http://127.0.0.1:3000/Emailer/index 测试您的应用程序。它显示以下屏幕,通过使用此屏幕,您将能够将消息发送给任何人。
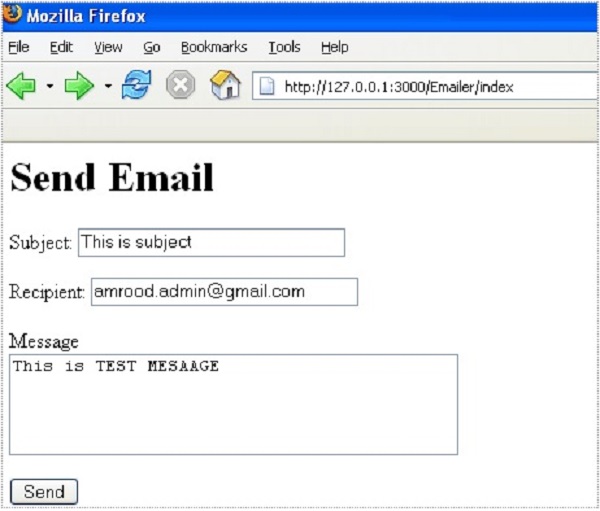
发送消息后,会显示短信——“消息发送成功”。
使用 Rails 发送 HTML 电子邮件
要以 HTML 形式发送邮件,请确保您的视图(.erb 文件)生成 HTML 并在您的emails/app/models/emailer.rb文件中将内容类型设置为 html,如下所示 -
class Emailer < ActionMailer::Base def contact(recipient, subject, message, sent_at = Time.now) @subject = subject @recipients = recipient @from = 'no-reply@yourdomain.com' @sent_on = sent_at @body["title"] = 'This is title' @body["email"] = 'sender@yourdomain.com' @body["message"] = message @headers = {content_type => 'text/html'} end end
有关ActionMailer的完整详细信息,请查看标准 Ruby 文档。