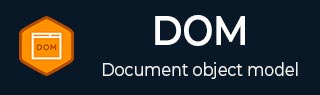
- XML DOM 基础知识
- XML DOM - 主页
- XML DOM - 概述
- XML DOM - 模型
- XML DOM - 节点
- XML DOM - 节点树
- XML DOM - 方法
- XML DOM - 加载
- XML DOM - 遍历
- XML DOM - 导航
- XML DOM - 访问
- XML DOM 操作
- XML DOM - 获取节点
- XML DOM - 设置节点
- XML DOM - 创建节点
- XML DOM - 添加节点
- XML DOM - 替换节点
- XML DOM - 删除节点
- XML DOM - 克隆节点
- XML DOM 对象
- DOM - 节点对象
- DOM - 节点列表对象
- DOM - 命名节点映射对象
- DOM - DOMI 实现
- DOM - 文档类型对象
- DOM - 处理指令
- DOM-实体对象
- DOM - 实体引用对象
- DOM - 表示法对象
- DOM - 元素对象
- DOM - 属性对象
- DOM - CDATASection 对象
- DOM - 评论对象
- DOM - XMLHttpRequest 对象
- DOM - DOMException 对象
- XML DOM 有用的资源
- XML DOM - 快速指南
- XML DOM - 有用的资源
- XML DOM - 讨论
XML DOM - 设置节点
在本章中,我们将研究如何更改 XML DOM 对象中的节点值。节点值可以更改如下 -
var value = node.nodeValue;
如果节点是属性,则值变量将是属性的值;如果节点是文本节点,则它将是文本内容;如果节点是一个Element它将是null。
以下部分将演示每种节点类型(属性、文本节点和元素)的节点值设置。
以下所有示例中使用的node.xml如下-
<Company> <Employee category = "Technical"> <FirstName>Tanmay</FirstName> <LastName>Patil</LastName> <ContactNo>1234567890</ContactNo> <Email>tanmaypatil@xyz.com</Email> </Employee> <Employee category = "Non-Technical"> <FirstName>Taniya</FirstName> <LastName>Mishra</LastName> <ContactNo>1234667898</ContactNo> <Email>taniyamishra@xyz.com</Email> </Employee> <Employee category = "Management"> <FirstName>Tanisha</FirstName> <LastName>Sharma</LastName> <ContactNo>1234562350</ContactNo> <Email>tanishasharma@xyz.com</Email> </Employee> </Company>
更改文本节点的值
当我们说 Node 元素的更改值时,我们的意思是编辑元素(也称为文本节点)的文本内容。以下示例演示了如何更改元素的文本节点。
例子
以下示例 (set_text_node_example.htm) 将 XML 文档 ( node.xml ) 解析为 XML DOM 对象并更改元素的文本节点的值。在本例中,将每个员工的电子邮件发送至support@xyz.com并打印值。
<!DOCTYPE html> <html> <head> <script> function loadXMLDoc(filename) { if (window.XMLHttpRequest) { xhttp = new XMLHttpRequest(); } else // code for IE5 and IE6 { xhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xhttp.open("GET",filename,false); xhttp.send(); return xhttp.responseXML; } </script> </head> <body> <script> xmlDoc = loadXMLDoc("/dom/node.xml"); x = xmlDoc.getElementsByTagName("Email"); for(i = 0;i<x.length;i++) { x[i].childNodes[0].nodeValue = "support@xyz.com"; document.write(i+'); document.write(x[i].childNodes[0].nodeValue); document.write('<br>'); } </script> </body> </html>
执行
将此文件保存为服务器路径上的set_text_node_example.htm (此文件和node.xml应位于服务器中的同一路径上)。您将收到以下输出 -
0) support@xyz.com 1) support@xyz.com 2) support@xyz.com
改变属性节点的值
以下示例演示了如何更改元素的属性节点。
例子
以下示例 (set_attribute_example.htm) 将 XML 文档 ( node.xml ) 解析为 XML DOM 对象并更改元素属性节点的值。在这种情况下,每个员工的类别分别为admin-0、admin-1、admin-2并打印值。
<!DOCTYPE html> <html> <head> <script> function loadXMLDoc(filename) { if (window.XMLHttpRequest) { xhttp = new XMLHttpRequest(); } else // code for IE5 and IE6 { xhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xhttp.open("GET",filename,false); xhttp.send(); return xhttp.responseXML; } </script> </head> <body> <script> xmlDoc = loadXMLDoc("/dom/node.xml"); x = xmlDoc.getElementsByTagName("Employee"); for(i = 0 ;i<x.length;i++){ newcategory = x[i].getAttributeNode('category'); newcategory.nodeValue = "admin-"+i; document.write(i+'); document.write(x[i].getAttributeNode('category').nodeValue); document.write('<br>'); } </script> </body> </html>
执行
将此文件保存为服务器路径上的set_node_attribute_example.htm (此文件和node.xml应位于服务器中的同一路径上)。结果如下 -
0) admin-0 1) admin-1 2) admin-2