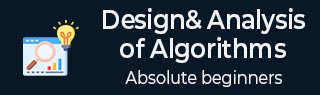
- 算法设计与分析
- 家
- 算法基础知识
- DAA - 简介
- DAA - 算法分析
- DAA-分析方法
- 渐近符号和先验分析
- 时间复杂度
- 马斯特定理
- DAA - 空间复杂性
- 分而治之
- DAA-分而治之
- DAA - 最大最小问题
- DAA-归并排序
- DAA-二分查找
- 施特拉森矩阵乘法
- 唐叶算法
- 河内塔
- 贪心算法
- DAA-贪婪法
- 旅行商问题
- Prim 的最小生成树
- 克鲁斯卡尔的最小生成树
- Dijkstra 的最短路径算法
- 地图着色算法
- DAA-分数背包
- DAA - 带截止日期的作业排序
- DAA - 最佳合并模式
- 动态规划
- DAA-动态规划
- 矩阵链乘法
- 弗洛伊德·沃歇尔算法
- DAA - 0-1 背包
- 最长公共子序列
- 旅行商问题| 动态规划
- 随机算法
- 随机算法
- 随机快速排序
- 卡格的最低削减
- 费舍尔-耶茨洗牌
- 近似算法
- 近似算法
- 顶点覆盖问题
- 设置封面问题
- 旅行推销员近似算法
- 排序技巧
- DAA-快速排序
- DAA-冒泡排序
- DAA——插入排序
- DAA-选择排序
- DAA——希尔排序
- DAA-堆排序
- DAA——桶排序
- DAA——计数排序
- DAA - 基数排序
- 搜索技巧
- 搜索技术介绍
- DAA - 线性搜索
- DAA-二分查找
- DAA - 插值搜索
- DAA - 跳转搜索
- DAA - 指数搜索
- DAA - 斐波那契搜索
- DAA - 子列表搜索
- DAA-哈希表
- 图论
- DAA-最短路径
- DAA - 多级图
- 最优成本二叉搜索树
- 堆算法
- DAA-二叉堆
- DAA-插入法
- DAA-Heapify 方法
- DAA-提取方法
- 复杂性理论
- 确定性计算与非确定性计算
- DAA-最大派系
- DAA - 顶点覆盖
- DAA - P 级和 NP 级
- DAA-库克定理
- NP 硬课程和 NP 完全课程
- DAA - 爬山算法
- DAA 有用资源
- DAA - 快速指南
- DAA - 有用的资源
- DAA - 讨论
设计与分析 提取法
Extract方法用于提取Heap的根元素。以下是算法。
Algorithm: Heap-Extract-Max (numbers[]) max = numbers[1] numbers[1] = numbers[heapsize] heapsize = heapsize – 1 Max-Heapify (numbers[], 1) return max
例子
让我们考虑前面讨论的相同示例。现在我们要提取一个元素。该方法将返回堆的根元素。

删除根元素后,最后一个元素将被移动到根位置。

现在,Heapify 函数将被调用。Heapify之后,生成如下堆。

例子
#include <stdio.h> void swap(int arr[], int i, int j) { int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } void maxHeapify(int arr[], int size, int i) { int leftChild = 2 * i + 1; int rightChild = 2 * i + 2; int largest = i; if (leftChild < size && arr[leftChild] > arr[largest]) largest = leftChild; if (rightChild < size && arr[rightChild] > arr[largest]) largest = rightChild; if (largest != i) { swap(arr, i, largest); maxHeapify(arr, size, largest); // Recursive call to continue heapifying } } int extractMax(int arr[], int *heapSize) { if (*heapSize < 1) { printf("Heap underflow!\n"); return -1; } int max = arr[0]; arr[0] = arr[*heapSize - 1]; (*heapSize)--; maxHeapify(arr, *heapSize, 0); // Heapify the updated heap return max; } int main() { int arr[] = { 55, 50, 30, 40, 20, 15, 10 }; // Max-Heap int heapSize = sizeof(arr) / sizeof(arr[0]); int max = extractMax(arr, &heapSize); // Extract the max element from the heap printf("Extracted Max Element: %d\n", max); // Print the updated Max-Heap printf("Updated Max-Heap: "); for (int i = 0; i < heapSize; i++) printf("%d ", arr[i]); printf("\n"); return 0; }
输出
Extracted Max Element: 55 Updated Max-Heap: 50 40 30 10 20 15
#include <iostream> #include <vector> void swap(std::vector<int>& arr, int i, int j) { int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } void maxHeapify(std::vector<int>& arr, int size, int i) { int leftChild = 2 * i + 1; int rightChild = 2 * i + 2; int largest = i; if (leftChild < size && arr[leftChild] > arr[largest]) largest = leftChild; if (rightChild < size && arr[rightChild] > arr[largest]) largest = rightChild; if (largest != i) { swap(arr, i, largest); maxHeapify(arr, size, largest); // Recursive call to continue heapifying } } int extractMax(std::vector<int>& arr, int& heapSize) { if (heapSize < 1) { std::cout << "Heap underflow!" << std::endl; return -1; } int max = arr[0]; arr[0] = arr[heapSize - 1]; heapSize--; maxHeapify(arr, heapSize, 0); // Heapify the updated heap return max; } int main() { std::vector<int> arr = { 55, 50, 30, 40, 20, 15, 10 }; // Max-Heap int heapSize = arr.size(); int max = extractMax(arr, heapSize); // Extract the max element from the heap std::cout << "Extracted Max Element: " << max << std::endl; // Print the updated Max-Heap std::cout << "Updated Max-Heap: "; for (int i = 0; i < heapSize; i++) std::cout << arr[i] << " "; std::cout << std::endl; return 0; }
输出
Extracted Max Element: 55 Updated Max-Heap: 50 40 30 10 20 15
import java.util.Arrays; public class MaxHeap { public static void swap(int arr[], int i, int j) { int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } public static void maxHeapify(int arr[], int size, int i) { int leftChild = 2 * i + 1; int rightChild = 2 * i + 2; int largest = i; if (leftChild < size && arr[leftChild] > arr[largest]) largest = leftChild; if (rightChild < size && arr[rightChild] > arr[largest]) largest = rightChild; if (largest != i) { swap(arr, i, largest); maxHeapify(arr, size, largest); // Recursive call to continue heapifying } } public static int extractMax(int arr[], int heapSize) { if (heapSize < 1) { System.out.println("Heap underflow!"); return -1; } int max = arr[0]; arr[0] = arr[heapSize - 1]; heapSize--; maxHeapify(arr, heapSize, 0); // Heapify the updated heap return max; } public static void main(String args[]) { int arr[] = { 55, 50, 30, 40, 20, 15, 10 }; // Max-Heap int heapSize = arr.length; int max = extractMax(arr, heapSize); // Extract the max element from the heap System.out.println("Extracted Max Element: " + max); // Print the updated Max-Heap System.out.print("Updated Max-Heap: "); for (int i = 0; i < heapSize; i++) System.out.print(arr[i] + " "); System.out.println(); } }
输出
Extracted Max Element: 55 Updated Max-Heap: 50 40 30 10 20 15 10
def swap(arr, i, j): arr[i], arr[j] = arr[j], arr[i] def max_heapify(arr, size, i): left_child = 2 * i + 1 right_child = 2 * i + 2 largest = i if left_child < size and arr[left_child] > arr[largest]: largest = left_child if right_child < size and arr[right_child] > arr[largest]: largest = right_child if largest != i: swap(arr, i, largest) max_heapify(arr, size, largest) # Recursive call to continue heapifying def extract_max(arr, heap_size): if heap_size < 1: print("Heap underflow!") return -1 max_element = arr[0] arr[0] = arr[heap_size - 1] heap_size -= 1 max_heapify(arr, heap_size, 0) # Heapify the updated heap return max_element arr = [55, 50, 30, 40, 20, 15, 10] # Max-Heap heap_size = len(arr) max_element = extract_max(arr, heap_size) # Extract the max element from the heap print("Extracted Max Element:", max_element) # Print the updated Max-Heap print("Updated Max-Heap:", arr)
输出
Extracted Max Element: 55 Updated Max-Heap: [50, 40, 30, 10, 20, 15, 10]